The ExecutorService Interface – Concurrency: Part II
The ExecutorService Interface
Table 23.2 lists the executor services provided by the factory methods of the Executors class. An executor service implements the ExecutorService interface that extends the Executor interface with methods that provide the following functionality:
- Flexibly submitting tasks to the executor service and handling the results returned (p. 1436)
In addition to the execute() method, an executor service provides the overloaded methods submit(), invokeAll(), and invokeAny() for submitting tasks. The Future<V> interface provides methods to handle the execution status and the results returned by tasks (p. 1435). The Callable<V> functional interface can be used to implement tasks that return a value (p. 1434).
- Managing the executor lifecycle
The ExecutorService interface provides methods to manage the lifecycle of an executor (Figure 23.2). Once an executor service is created, for example, by static methods of the Executors class, it is in the RUNNING state, ready to accept tasks for execution. As an executor service is a precious resource, it must be shut down by calling its shutdown() or shutdownNow() method. An executor service in the SHUTDOWN state does not accept new tasks, which are discarded. However, although the executor service might be shut down, some of its threads might still be executing tasks and other tasks might be waiting to be executed. Only when all tasks have completed does the executor service transition to the TERMINATED state. The methods isShutdown() and isTerminated() can be used to check if the executor service is in the SHUTDOWN or TERMINATED state, respectively. The method awaitTermination(), called on an executor service after it is shut down, can be used to wait for threads to complete their tasks. See Example 23.1, p. 1429.
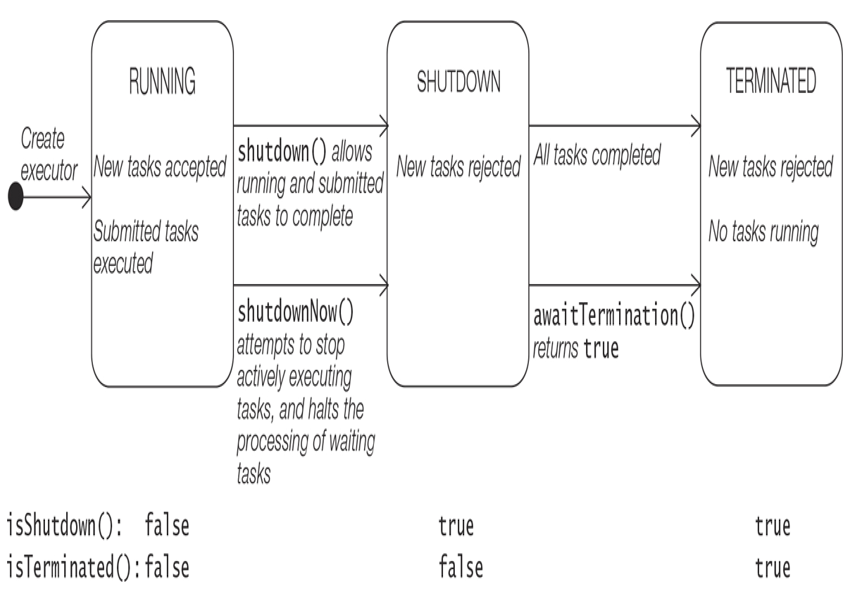
Figure 23.2 Executor Service Lifecycle
Following are selected methods of the ExecutorService interface for shutting down and terminating an executor service:
void shutdown()
Starts an orderly shutdown in which previously submitted tasks are executed, but new tasks are rejected. This method does not wait for already submitted tasks to complete execution. A shutdown request on an executor that is already shut down has no additional effect.
List<Runnable> shutdownNow()
Attempts to stop all actively executing tasks, and halts the processing of waiting tasks. It returns a list of the tasks that were awaiting execution.
This method does not wait for actively executing tasks to terminate.
This method at best attempts to stop processing of actively executing tasks— however, there are no guarantees. Typically, task cancellation will be triggered via Thread.interrupt(), so any task failing to respond to interrupts may never terminate (§22.4, p. 1393).
boolean isShutdown()
Returns true if this executor has been shut down—that is, a shutdown request has already been invoked on the executor.
boolean awaitTermination(long timeout, TimeUnit unit)
throws InterruptedException
Blocks until one of these events occurs first: All tasks have completed execution after a shutdown request, or the timeout occurs, or the current thread is interrupted. This method should be called after calling the shutdown() method.
boolean isTerminated()
Returns true if all tasks have completed following shutdown. It never returns true unless a shutdown request has already been invoked on the executor.